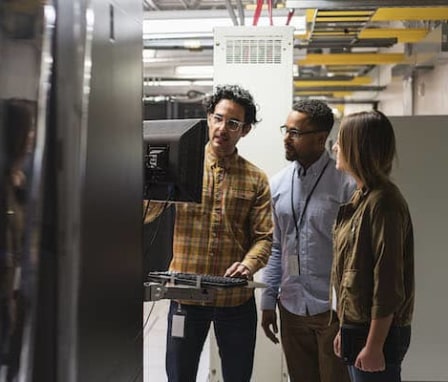
Skilled developers are in high demand. The Bureau of Labor Statistics predicts employment for software developers will grow 22 percent between 2012 and 2022 primarily due to the increasing demand for new applications, from mobile to healthcare to security software. While there are a number of coding languages for aspiring and current developers to learn, Ruby on Rails has inched its way towards the top of the list over the last few years and there are now many job opportunities specifically for Ruby on Rails programmers.
The following guide will explore Ruby on Rails, from its philosophy and conventions to its uses and benefits. Also included are detailed information and expert-vetted resources on how and where beginner and advanced programmers can learn the skills necessary for a successful career as a Ruby on Rails developer.
Understanding Ruby, Ruby on Rails, and RubyGems
Most people who are not familiar with Ruby on Rails often confuse the terms “Ruby” and “Ruby on Rails”. Ruby is a general purpose programming language, invented in 1995 by Yukihiro ‘Matz’ Matsumoto. Ruby on Rails – sometimes called just “Rails” – is an open-source web application framework written in Ruby and introduced by David Heinemeier Hansson in 2007. It is used to make web applications. Though the two are separate – one is a language, while the other is the framework for the language – they can be learned simultaneously.
Ruby
Ruby is used for all purposes, from building desktop applications to building command line applications. It is an interpreted language, which means that the interpreter runs the code on fly, and you don’t have to compile the code to a binary file. It’s also an object-oriented language. Some argue that it is the purest object-oriented language because everything in Ruby is an object.
Rails
Rails is based on a popular Model View Controller or shortly known as MVC pattern, in which the application is divided into three basic components. Models deal with the data, and all the business logic needed to read and write that data. Views are used to build the presentation or User Interface of the application. Controllers work as a bridge that connects two components together. Models and Views can’t communicate directly with each other and, therefore, always need a Controller for communication. In controller, a programmer defines different methods – a chunk of code that one can use multiple times by calling that method – to perform tasks.
Here’s how a typical model looks like in Ruby:
class User < ActiveRecord::Basevalidates :email, presence: trueafter_save :send_welcome_emailend
The string above is an example of validation, which is used often in Rails. Validation is a set of conditions that a user must fulfill before he or she tries to do something. For example, if one wants to save a user to a database, one should specify email for the user, because there is applied validation for it.
In this particular example, a user cannot be saved in a database until an email is associated with that user. `after_save` is a callback for each time a user is saved in the database. A callback is a place where you can write stuff, and it executes when a particular event happens. For example, `after_save :send_welcome_email` means that each time, a user is saved, the method `send_welcome_email` will be invoked.
The following is a snippet from a Controller file from a Ruby on Rails project:
class UsersController < ApplicationControllerbefore_action :authenticate_user!
def create@user = User.new(params.permit(:user).require(:email))if @user.saveredirect_to root_pathelserender ‘new’endendend
In this example the only action is `create`. If the user is saved, the request is redirected to `root_path`. If the user isn’t saved, it renders something called `new`.
The last component is View, the presentation layer that is visible to the user. Here’s a snippet of code that shows all the user records saved in the database:
<% @users.each do |user| %><p class=”user_email”>Email: <%= user.email %></p><% end %>
A typical view file has an extension of `.html.erb` in which `erb` stands for Embedded Ruby, that means that you can insert Ruby code inside your view file, which is what is being done in the example snippet above.
`<% %>` is a place to insert Ruby code. This executes the Ruby code and doesn’t print the output, whereas `<%= %>` executes the Ruby code and also prints it.
RubyGems
RubyGems (singular: RubyGem) are similar to libraries in other coding languages. They are a simple way to package code for the purpose of re-usability. Gems are available for almost every popular task: If you want to use authentication in your web application, you may use **devise**, **sorcery**; if you want to leverage the power of Google Maps in your web application, you may use **geocoder** gem; if you want to print the data in your application to PDF format, you may use the gem **pdfkit**. Programmers also have the option of creating their own gem.
When you create a Rails project, a file called **Gemfile** gets produced along the way. This file keeps record of all the gems used in your project. If you want to use a new gem, you have to write: `gem ‘name_of_new_gem‘`, then run the command `bundle install` to install the gem. From there, you will be able to use all the code that was packaged in that particular gem. There are hundreds of gems available at https://rubygems.org.
Top Online Programs
Explore programs of your interests with the high-quality standards and flexibility you need to take your career to the next level.
Uses and Benefits of Ruby on Rails
Usually, a Ruby on Rails developer is responsible for handling data at the backend, which means he or she deals with databases, servers, and scaling which is when one has to make an application in such a way that millions of users can use it at the same time without having delays or crashes. Rails is not limited to web applications – it is also extensively used for building web services. Developers can build a complete application-programming interface (API) in Rails. More and more programmers are joining the growing community of Rails development. Many major companies are also investing resources in Rails because it takes little time to develop applications in this framework. Additional benefits of Ruby on Rails include:
Cutting edge technology
Rails uses cutting-edge technologies, which means developers get to work with the latest technologies and patterns. As of Rails 4.2, if you create a new project in Rails, it uses SASS (Syntactically Awesome Style Sheets) and Coffeescript instead of CSS and JavaScript. SASS is a way to write better, more modular, and reusable CSS. Similarly, Coffeescript allows one to write less lines of code to accomplish the same task that would have required more lines of code when using plain JavaScript.
Testing
Rails encourages developers to test their code. If you generate a model or a controller in your Rails project, Rails also generates test files. This is called TDD or test driven development, and it is something that makes your code more secure. It also makes it easy to add new features to your application.
Speed
Rails uses MD5 hash (a guaranteed unique and random 40 character key) with assets like JS, CSS files, and images, which means once a browser has cached these files, the server won’t serve these files next time. This reduces the loading time of web applications.
Some of largest sites that are leveraging the power of Rails include:
An online code collaboration application where thousands of developers can share their code.
Yammer is a web application used for intra-organization communication. This is an example of enterprise social software.
Scribd is a digital platform for e-books, audio books and comic books.
An ecommerce platform written in Ruby on Rails for the purpose of selling goods and building brands and customer relationships.
A web-based project management tool that helps users manage projects efficiently.
Learning Ruby on Rails
A little background in programming, a keen interest to learn Ruby on Rails, and some thoughtful planning can make it easy and fun to learn this coding language. Below is an overview of the different ways individuals can learn Ruby on Rails:
Colleges and Universities
Pursing a computer science degree from a four-year college or university is a great way to not only learn Ruby on Rails, but also build a solid foundation in computer science. There are several core courses that CS students must take, such as object-oriented programming, data structures, algorithms, object-oriented design, and databases, which all help aspiring programmers understand the basics of web application. Programmers can build a fully functional web application without having a CS degree, but if you really want to understand what exactly is going on under the hood, a degree in computer science will deepen your understanding of key areas such as databases, data structures, object-oriented programming, and artificial intelligence.
Online tutorials
Every student learns in a different way. While a CS degree is a great way to get a deep understanding of programming, this option can be expensive and going to college for four or more years isn’t doable for everyone. For those looking for an alternative, online tutorials are an ideal route. Online tutorials allow students to learn anywhere, anytime and are often more affordable than pursuing a full CS degree. Below are examples of a few online sites where aspiring Ruby on Rails developers can learn all they need to know, from the comfort of their own home.
Founded in 1995, this is an online learning company that offers high quality courses in business, software, technology, and creative skills. Users can sign up for a free 10-day trial and then continue their membership for $25/month.
Learning Rails requires a familiarity with HTML, CSS, JavaScript, and Ruby. With that in mind, below are a few popular Lynda.com courses for aspiring Rails developers:
- HTLM Essential Training – Covers the basics of HTML and CSS
- Foundations of Programming: Fundamentals – An ideal course for beginners with very little to no background in programming
- Foundations of Programming: Web Services – An overview of web services, what they are and when to use one over the other
- Ruby on Rails Essential Training – After learning the basics of HTML, CSS, and JavaScript, this course takes students into actual Rails development
Code School is an online learning platform specifically for aspiring and current developers who lack the time, financial backing, and/or flexibility needed to pursue a full computer science degree. Students can sign up for a free account or get more access by starting a no contract membership for $29/month.
Code School offers several courses on Ruby on Rails, including:
- Try Ruby
- Rails for Zombies Redux
- Rails for Zombies 2
- Ruby Bits
- Ruby Bits Part 2
Unlike other video tutorial sites, Codecademy is a site where students can start learning by jumping right in and writing code. This is a great tool to use in combination with other tutorial sites. The courses that are offered for Ruby on Rails include:
- Make a Website
- Learn Ruby on Rails
- Ruby on Rails: Authentication
Coding Bootcamps
Some students find it hard to learn via online tutorials and, therefore, would prefer to work with an instructor to learn Ruby on Rails. For such students, coding bootcamps may be the ideal fit. Bootcamps are short-term, fast-paced programs that provide a “crash course” on Ruby on Rails. They can be very demanding and intense, but will cover everything a student needs to know in order to land a job in the development world and become a successful programmer. Below are some of the most popular bootcamps for Ruby on Rails:
The guys at Big Nerd Ranch offer a complete course for learning and exploring Rails that they call Ruby on the server. It’s a five day coding session, in which expert authors will guide you through the major components of Rails, building web services, exploring the Rack middleware, and much more.
DevBootcamp provides you different training sessions ranging from 9 weeks, 9 to 15 weeks, to even ongoing career support. Based in Chicago, New York, and San Francisco, the company offers teaching on Ruby, as well as JavaScript, SQL, and HTML/CSS. As a student, you will receive daily challenges, and at the end, must complete a big final project. By constantly focusing on applying what you have learned, you can get a lot out of it. Teachers and mentors are always around, so you can have a professional review of your code and provide you with feedback.
This is a four-month coding bootcamp, in which students will be given a lot of lessons and assignments focused on production ready projects. It is a great way to get hands-on experience with Rails. Students will learn the core principles of building web applications.
The Philosophy of Rails
The popularity of Rails has grown tremendously because of its guiding principles. Below is an overview of the main philosophies:
Don’t Repeat Yourself (or DRY)
This is the core principle for Rails. The idea is to reduce code repetition within a system, which makes the code manageable, efficient, and flexible. Rails gives developers the following ways to make sure they do not have to repeat themselves: Application Controller
If a function is being used in most of the controllers, programmers can take that function and write it down in Application Controller. The functionality then becomes available to the rest of the controllers. Concerns
If models share a lot of common behavior, programmers can extract the common behavior, wrap it in a module, and put that module in the `app/models/concerns` directory so that all the code will be stored in one place and other models can use easily use that behavior.
DRY code also allows programmers to make fewer changes. For example, if the common code is written in one place, you would change the code in that one location. The changes would then be reflected throughout and you wouldn’t have to manually modify the behavior in each file.
Convention over Configuration (or CoC)
This principle is designed to reduce the time and effort needed for a developer to develop something. There are several conventions that Rails follows, including:
A model name should always be singular, start with an uppercase letter, and follow the **camelCase** convention (joining the two words together and making the first letter of each subsequent word uppercase). The name of the file that contains the model class should be singular as well, but all lowercase, and should follow the snake naming pattern (where words are joined together with an underscore). For example, `CurrencyRate` is the name of the model and `currency_rate.rb` is the name of the file.
Table names that are actually stored in the database are always plural, and follow the snake naming pattern. For `CurrencyRate` model, it should be `currency_rates`.
Similarly, the name of the controller should be `CurrencyRatesController`: plural, starts with an uppercase letter, follows the **camelCase** convention, and ends with the word `Controller`, and the name of the file that contains the controller should be `currency_rates_controller.rb`. It is worth mentioning that in other frameworks like Sinatra, conventions are not imposed the way they are imposed in Rails. For example, you can name your controller whatever you like, but as a result, it becomes difficult for other developers to understand your code.
Conventions make it easier for other developers to follow your code. Additionally, the more conventions are there, the less code to write, which saves time and effort.
Fat models, skinny controllers
This motto refers to the code structure for the M and C parts of MVC. Models should contain more code and, therefore, are “fat”, whereas controllers contain less code and are, therefore, “skinny”. Controllers are merely for the purpose of connecting Models and Views. And it shouldn’t take more than a few lines of code to connect the two parts. Models, on the other hand, need to do a lot of business logic and database things, which requires them to contain a lot of code.
Resources for Beginners
For those looking to learn more about the basics of Ruby on Rails, here is a list of beginner resources to help get you started:
Agile Web Development with Rails 4 (Facets of Ruby)
A perfect book for a beginner. What’s best about this book is that it teaches you every aspect of Ruby on Rails in one single book. Things like TDD, BDD, Git, and others; best for you if you want to get it all in one book.
Codeschool Rails for Zombies Redux
Packed with fun, badges, and challenges, this course covers the most basic aspects of Ruby on Rails. Perfect for anyone who is just getting started.
Official guide by Ruby on Rails community, great if you want to get it all: What is Rails, how do you install it; you get the idea.
Getting Started with Ruby on Rails
An excellent course that covers the basics of all the tasks that you may need to do in Ruby on Rails. A relatively short course (2.4 hours), good for a person who quickly wants to get up and running with Rails.
A codecademy course, it will take approximately 5 hours to cover this course. Great for someone who learns by doing as this course has no instructional videos, and you are supposed to learn by actually typing the code in your browser.
Lynda.com Ruby on Rails 4 Essential Training
Starts with the basics, and at the end, author Kevin Skoglund builds a real world web application. Though a long one, nearly of 12 hours, but worth the effort.
Programming Ruby 1.9 & 2.0: The Pragmatic Programmers’ Guide (The Facets of Ruby)
Ruby is crucial to any developer who wants to take his Rails knowledge to the next level. This book covers all you need to know about Ruby, and RubyGems.
Not a single course, but a track that consists of many courses. For the novice looking for the basics of web development.
Ruby on Rails 4: Getting Started
Brought by Pluralsight, this course covers the basic of Rails, and also dives into the topics like Assets Pipeline, and deployment.
Written by the creator of Ruby himself, this book aims on getting started with Ruby and its libraries. This is relatively a short book, but perfect if you want to get on to the next level.
Advanced Ruby on Rails Resources
Once students have the basics of Rails down, those who want to move on to more advanced learning will find the following list of resources helpful.
Metaprogramming Ruby 2: Program Like the Ruby Pros (Facets of Ruby)
Ruby is a rich language, and it also supports meta programming, that means you can build the functionality at the run time, and that’s what Rails does. So this book teaches all you need to discover about the world of metaprogramming in Ruby as well as in Rails.
Practical Object-Oriented Design in Ruby: An Agile Primer
This book isn’t intended to teach you Ruby, but this book teaches you the most important thing using Ruby: Object-oriented design principles. One of the classical book on this subject, the author Sandi Metz does an excellent job of explaining all the stuff into a book of nearly 200 pages.
The best course to learn all the new features introduced in the newest major release of Rails as of this writing: Rails 4. You will get to learn `ActionController::Live`, memcache client and more in this course.
Though not a very advanced course, but great to refresh the intermediate stuff regarding Ruby on Rails. This course includes all you need to build a pretty complex Rails application.
Ever wondered how does Rails handle the process of rendering for you, if yes, this is the perfect course to check out. If you want to dive into the internals of Rails, this may be the first step to that journey.
If you want to learn advanced Ruby at the comfort of your browser, these two are the perfect courses for you to take. These courses contain videos as well as challenges for strengthening your knowledge about Ruby that will automatically enhance your understanding of Rails.
Ruby MVC Framework From Scratch
This course builds a Rails like framework from scratch to help you understand how the process of processing the request in Rails happens. This is relatively a short course, nearly of 2 hours, but great if you want to explore the Rails internals.
If you are into building the web services stuff along with using test driven development in Rails, this is the perfect course for you to get started with writing APIs in Ruby on Rails.
RSpec is a great way to write tests for your Rails application. This course covers all you need to learn about RSpec and Factories to effectively write tests. RSpec is preferred in favor of Minitest in Rails community.
It is the book that every serious Rails developer out there should read, as it contains all the advanced stuff regarding different aspects of Ruby on Rails. Consisting of almost 900 pages, this mighty book introduces you not only the advanced stuff, but also the best ways to use the advanced things in Rails.
Ruby on Rails Careers
Rails is a top choice for those looking to land a job as a web developer, particularly for those most interested in joining a startup since Rails is one of the most popular programming languages for backend software development. Backend developers work on the things users don’t see – the things that happen on the server side. Specific responsibilities and projects for Ruby on Rails developers will vary, depending on the company and its backend development needs, but in general these developers will work with web servers, databases, and applications.
Below is a list of example job titles for Ruby on Rails developers:
- Ruby on Rails Developer
- Full Stack Rails Developer
- Web Developer
- Junior Developer
- Mid-Level Developer
- Senior Developer
- Software Engineer
Recommended Reading
Take the next step toward your future.
Discover programs you’re interested in and take charge of your education.